For the latest lab in my Open Source Development course, our professor designed a bridge troll project which maps bridge data in Ontario. The premise is that there are trolls hiding beneath bridges, and the app will use geolocation to find out your current position, and mark the bridges in your surroundings for possible troll sightings.
In order to run the app to test things out, I had to fork the repo into my Github account, and then clone to my local machine. However, as the upstream repo got more and more updated, I found myself having to make pulls to the upstream, and then push those updates into my origin. This made me wonder if there was another way to keep the forked repo in my own account automatically updated to keep in sync with the upstream repo. Nonetheless, since I am still learning to use Git properly/intuitively, I found myself having to start fresh several times, wiping up my local repo and re-cloning from either upstream or origin.
Frameworks used by bridge-troll:
After downloading the repo, I had to use npm to build the app. This was very smooth and painless. Some of the packages the project used include: babel, parcel and prettier. Babel is a transpiler which can take javascript code based on the latest standards, and transform the code into browser compatible javascript. This allows programmers to code using the latest javascript without having to worry about browsers not being able to render it. Our prof compared it to the Tower of Babel mythology that was used to explain why we have so many spoken languages today.
Parcel is a bundler that groups assets like CSS, images, html and javascript files together and sorts out the dependencies. This allows for easier importing around the code, translates the require()
statements URLs so that the browser can render it. The main feature for Parcel over Webpack is that Parcel does not require configurations, because it supports CSS, HTML, file assets and JS out of the box. While trying to understand what a bundler was, I found this blog which explained the purpose of bundlers like webpack.
Another module the project used was Prettier. This allowed the code to have consistent formatting by automatically reformatting the code to follow their style. The modules allows a project with many contributors to end up with code that follows a consistent style.
The task at hand:
One of the feature request for this project was to allow for changing of themes for the map that is displayed. The goal was to use a darker palette for the maps during night time and to use a lighter, more colourful palette during daytime instead. To achieve this goal, we need 3 things:
- Calculate the times for sunrise and sunset
- Changing tile sets used in the map
- Changing the icons used in the map
To calculate the sunrise and sunset times, I had imported an additional module into the project called sunCalc. This module calculates all relevant times such as sunrise, sunset, dawn, night and golden hours when provided a latitude and longitude. Since we are concerned with changing to a darker palette when the sun goes down, the suncalc times that are relevant are the sunset and sunrise hours. We would want to change to a darker theme after the sun goes down, and before the sun rises. Below is a screenshot of the code I wrote to determine when to switch into a dark theme:
The next thing is to figure out how to change the tile sets. From looking at the code, I realized the tile sets are changed in base-ui.js (formerly map.js). From the description in the feature request, I also know that the project uses leaflet to import tile sets used in the mapping. All I had to really do is browse through a collection of tile sets here, and select the tile sets I would like to use. Afterwards, I used the manageViewMode module I coded to get the mode I should be displaying, and feeding the corresponding url for the tile set I would like to display into a variable called tileUrl. Below is the relevant code I used to change the tile set in base-ui.js:
Lastly, I would like to change the icons into the white coloured version during nightime. The only icons I changed were the locked, unlocked, and location icons. Icons such as the map icon were left unchanged. To do this, I searched and downloaded each respective icon from Material Icons and added the icons to the icons folder of the project. Afterwards, I modified each icon’s file path such that it was dependent on the display mode in svg-marker.js file.
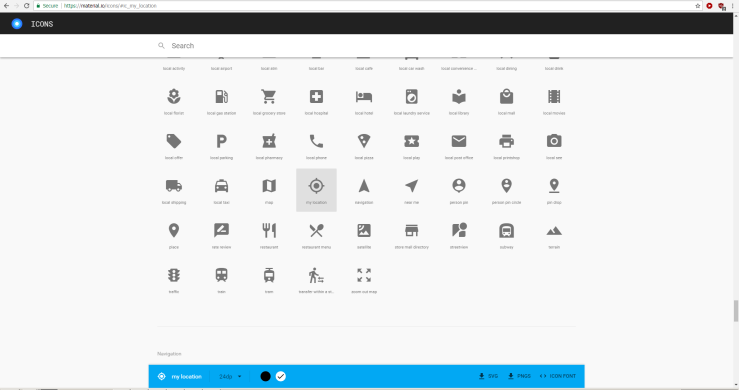
After making these changes, I uploaded my fix onto my GitHub repo, and then submitted a pull request into the bridge-troll project.
Here are some screenshots of the final look:
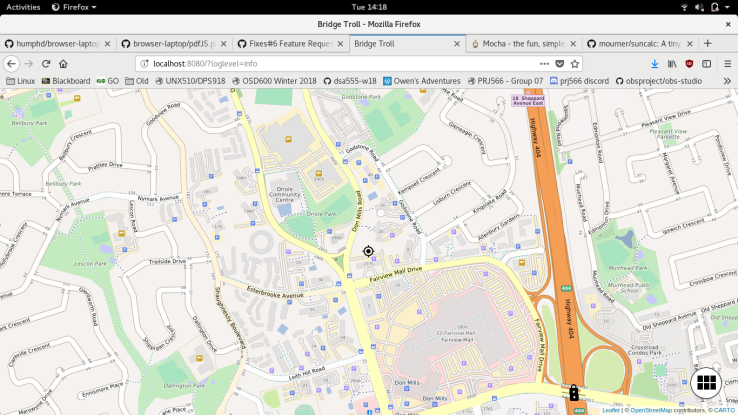
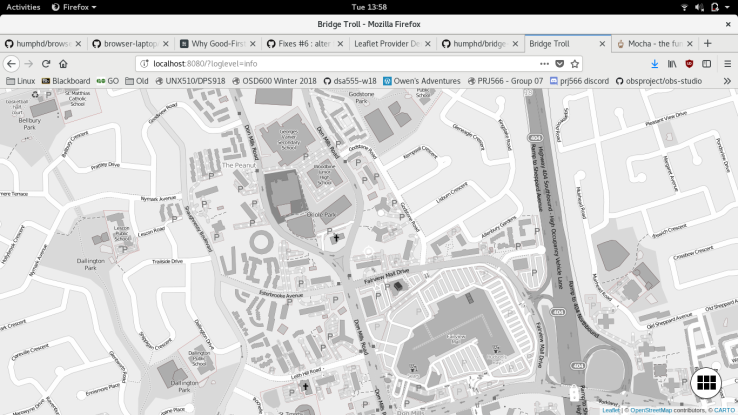
Overall experience:
The majority of the difficulties from implementing this feature came from my lack of javascript knowledge. I have not done much work in javascript since over a year ago where I had an introductory course into js, css, and html. My lack of proficiency in js not only made it very difficult to understand the code used in the project, but I found myself slowly reacquainting to basic js syntax as I was coding the new features. This lengthened process of implementing the feature by much longer than necessary.
Another difficulty I had was from the windows environment. Even though Git changes the line endings for the code during checkouts, the eslint was giving me issues when coding in windows. As a bypass, I had to switch to my Fedora laptop to do most of the coding instead.
The last difficulty of note is the testing of this application. At the beginning of the project, I had not know much about conditional debugging, and the use of log levels to reveal the testing statements made. As a result, I spent a lot more time guess and checking to see which statement was breaking the app. After being shown how to use log.info('Some useful test statement here')
, I was able to display my test statements passing the parameter ?loglevel=info
in the url. This revealed important facts such as the current time or the sunrise times during the execution of the app, which simplified the debugging process for me.
The pull request I submitted can be found here.